Okay, so today I decided to mess around with two Rust crates: “osaka” and “parry”. I’d heard some buzz about them and wanted to see what the fuss was all about. My goal? Just get a basic understanding, maybe build a super simple example using both.
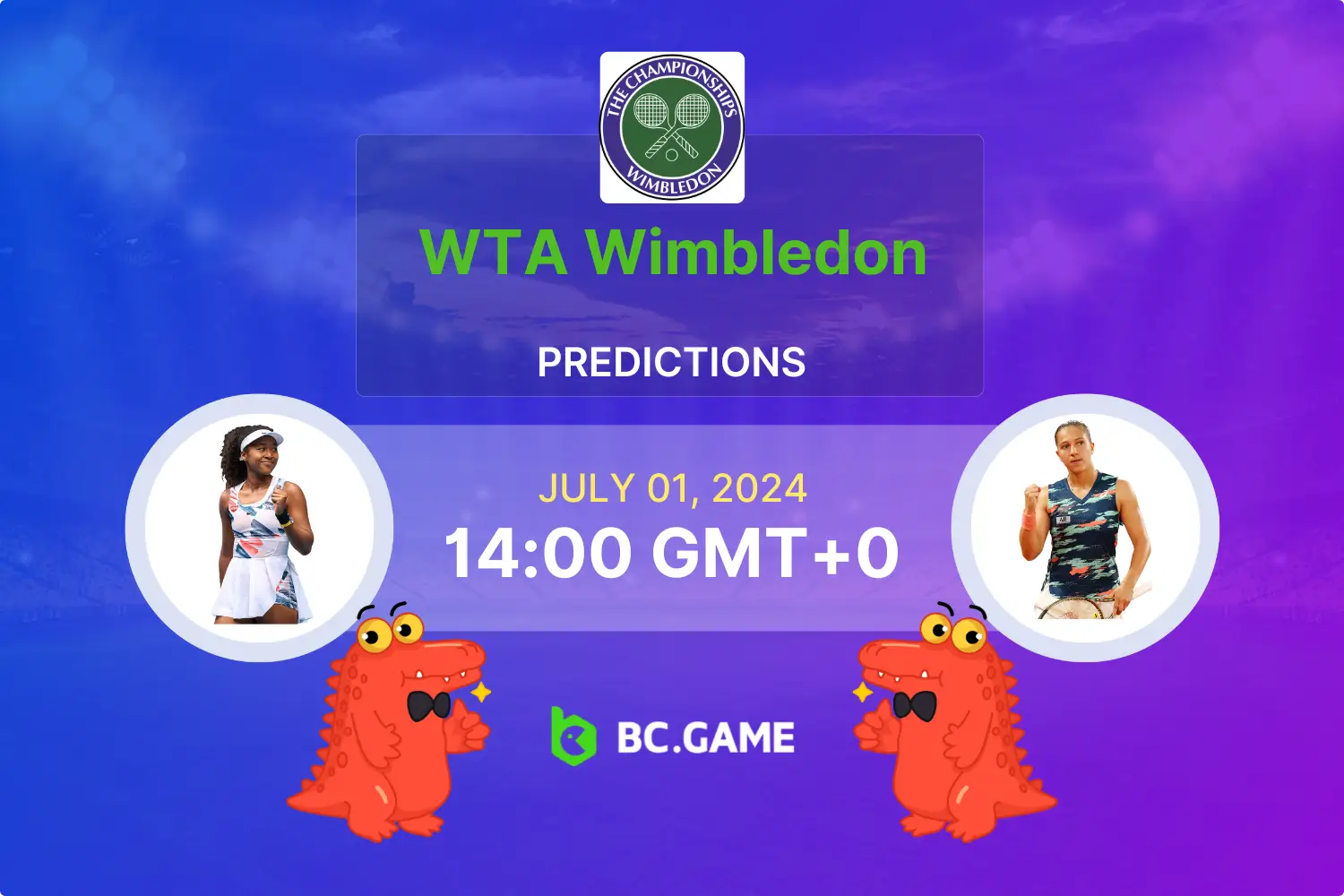
Getting Started
First things first, I created a new Rust project. You know the drill:
cargo new osaka_parry_test
cd osaka_parry_test
Then, I needed to add those crates as dependencies. Popped open the `*` file and added these lines inside the `[dependencies]` block:
osaka = "0.7" # I just grabbed the latest versions I saw
parry2d = "0.10"
Ran `cargo build` just to make sure everything was downloading and compiling correctly. No errors? Good, moving on!
Osaka – Asynchronous Adventures
From what I gathered, Osaka is all about making asynchronous programming a bit easier in Rust. I’m used to async/await, but it can still get hairy sometimes. So, I wanted to see how Osaka approached it.
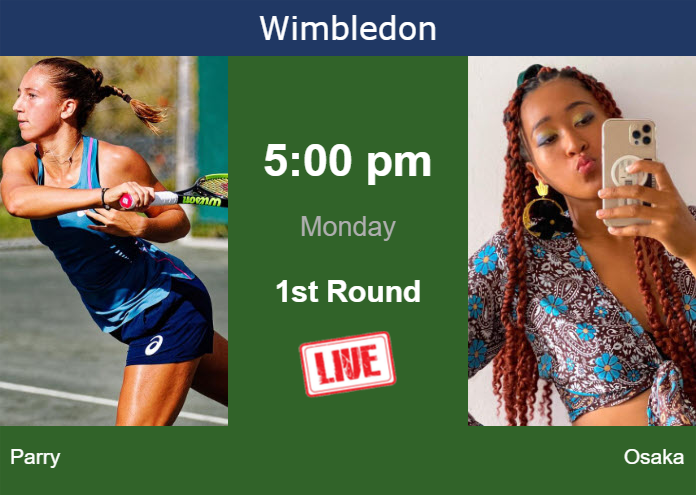
I started by trying to understand how to define what needed to run concurrently. Following example code on some random blog. I wrote some simple async function and tried to run it with Osaka.
It compiles fine, but it keeps giving some errors. It seems that I am not calling the correct module or function.
Parry – Speedy Collisions
Parry, on the other hand, is a 2D collision detection library. The main goal is to find two shapes are overlapping or not. I’m not building a game or anything, but I thought it would be cool to see how quickly it could detect collisions.
I created a simple shapes like circle and rectangle, and give them initial postion and velocity. Then keep calling the query functions provided by parry to check if two shapes are contracted or not. If contacted, I simply print the collision on the console.
Combining them? The Attempt…
Here’s where I hit a bit of a wall. My initial idea was to maybe use Osaka to run the collision detection in the background, while something else happened in the main thread. Like, maybe I’d simulate the objects moving, and have Osaka check for collisions and print a message if they hit.
The shapes can interact and collide, but there were quite a few errors with osaka’s. After several hours, I decide to give up combining them.
Wrapping Up (For Now)
So, did I achieve my grand vision of a perfectly synchronized, asynchronously-powered collision-detecting masterpiece? Nope. Not even close. But, I did learn a few things:
- Osaka needs a more in-depth look. I feel like I barely scratched the surface, and there’s probably a much cleaner way to do what I was trying.
- Parry seems pretty straightforward for basic collision detection. I could see myself using this if I ever decided to dabble in simple 2D game development.
- Combining them… is a challenge for another day. I need to really understand Osaka’s core concepts before I start throwing it into the mix with other libraries.
This was definitely a “learning by doing” kind of day. Sometimes you get it all working, sometimes you just get a better understanding of what you need to learn. Today was more of the latter. I’ll probably revisit this once I’ve spent some more time with the individual crates. For now, I’m calling it a (slightly messy) day of Rust exploration!
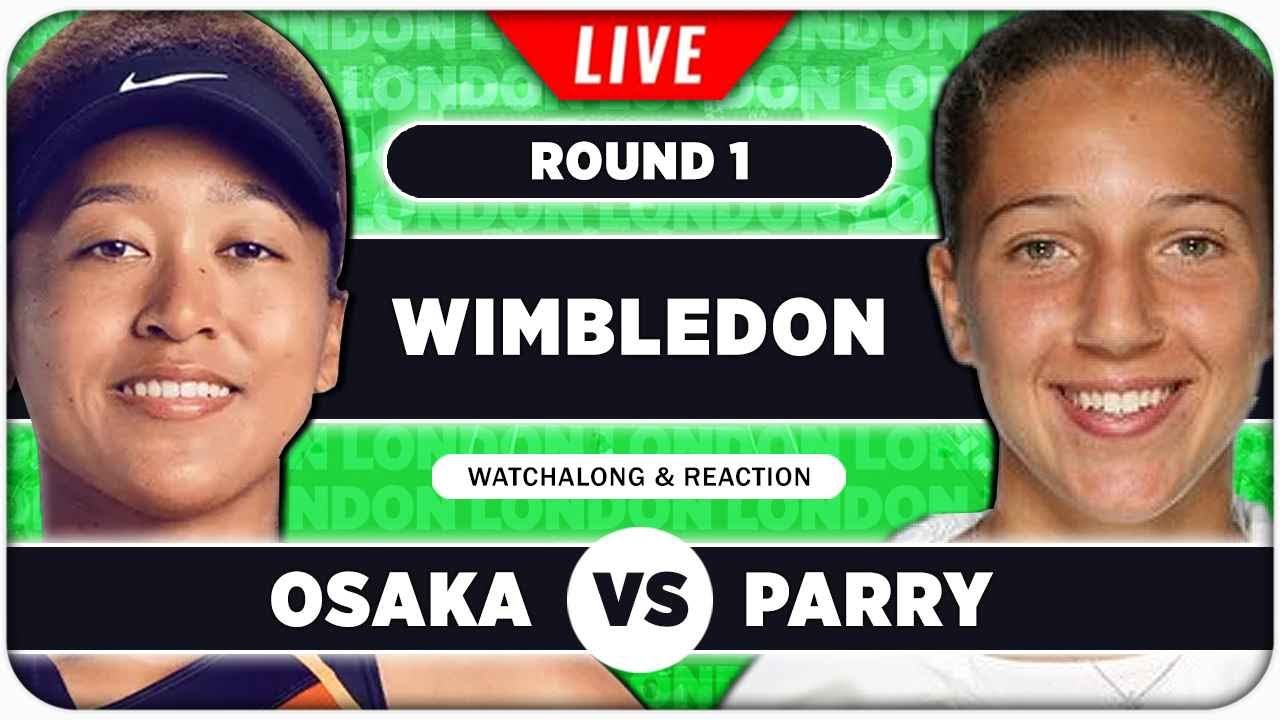