Okay, here’s my blog post about tackling Surface SLAM for the first time, written in a casual, experience-based style:
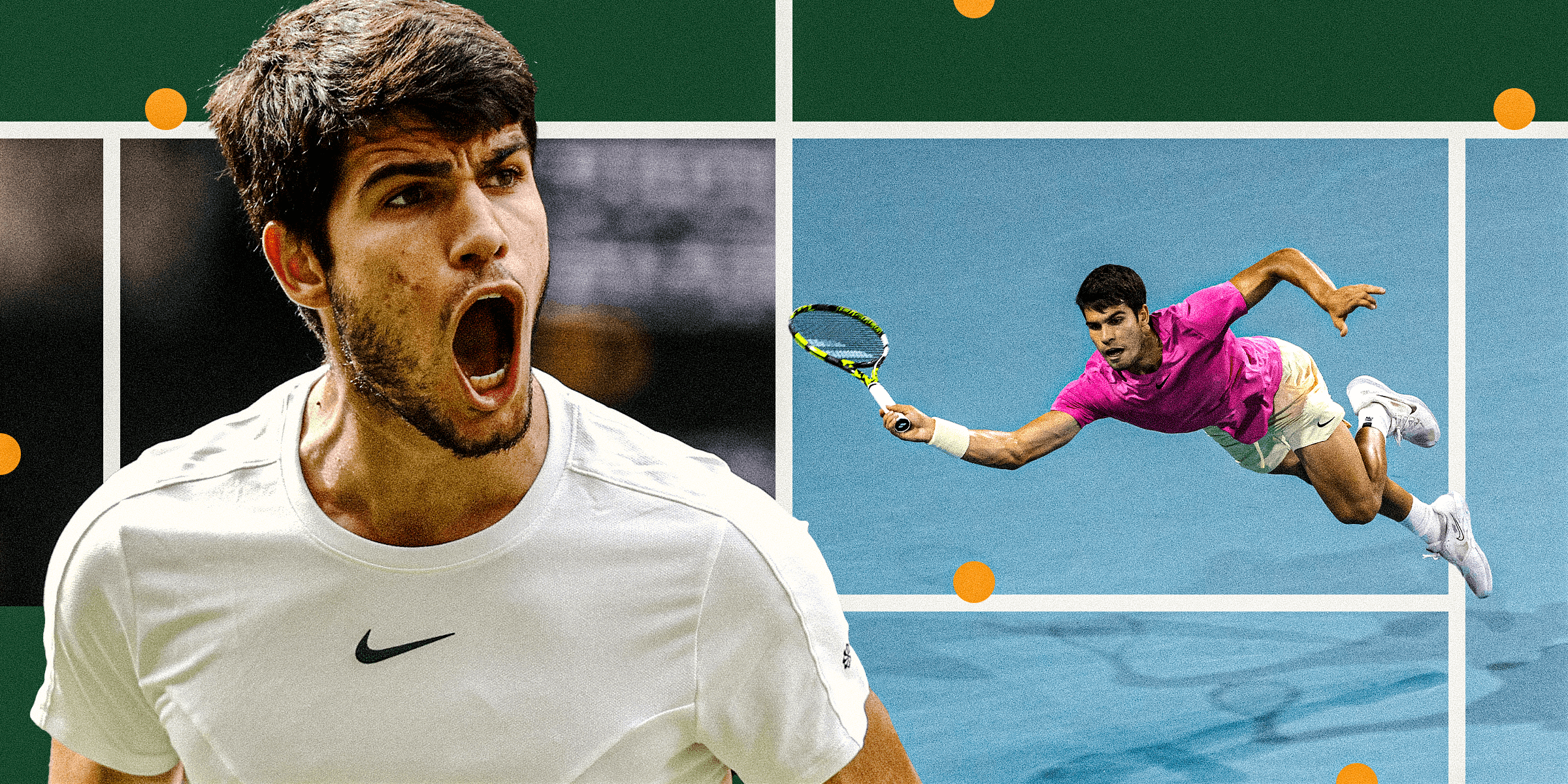
Hey everyone! So, I finally dove into Surface SLAM. I’d been putting it off, intimidated by all the jargon, but honestly, it wasn’t as bad as I thought. Here’s how it went down.
First off, I grabbed the data. I found a dataset online – some pre-recorded sequences from a depth camera moving around a room. Nothing fancy, just your basic RGB-D stuff. I made sure it was in a format I could easily load into my Python environment. Took a bit of digging to find the right libraries, but nothing too crazy.
Next, I started simple. I wasn’t trying to build the next HoloLens, just wanted to get something, anything, working. I focused on ICP (Iterative Closest Point). I figured if I could get two point clouds to align, I’d be on the right track. So, I found a basic ICP implementation online. There are tons out there, some are better than others, but for a first pass, anything works.
Then came the fun part – debugging! The initial ICP result looked like a total mess. The point clouds were all over the place. It took a while to figure out what was going wrong. Turns out, the initial guess for the transformation matrix was super important. If the two point clouds started too far apart, ICP would just get stuck in a local minimum. So, I added a small amount of smoothing using some of the numpy functions.
So, I tried again, and boom! The point clouds aligned much better. Still not perfect, but a massive improvement. I could actually see the shape of the room starting to emerge. This was enough to motivate me to keep going.
Now, I wanted to build a map. I created a loop that iterated through all the frames in the dataset. For each frame, I aligned it to the previous one using ICP. Then, I merged the transformed point cloud into a global map. This is where things got slow! Merging thousands of points takes time. I quickly realized I needed some kind of point cloud filtering to keep the map from exploding in size.
So, I added a voxel grid filter. This basically downsamples the point cloud by replacing all points within a small cube (a voxel) with a single point. It significantly reduced the number of points and sped things up. The map still drifted over time, but hey, that’s SLAM for you.
- Grabbed data
- Implemented ICP
- Debugged initial alignment issues
- Built a basic mapping loop
- Added a voxel grid filter
The final result wasn’t pretty. The map was a bit wobbly and had some noticeable drift, but you could still make out the general shape of the room. More importantly, I learned a ton! I now have a much better understanding of how Surface SLAM works and what the key challenges are. Next up: loop closure and more advanced filtering techniques. Stay tuned!
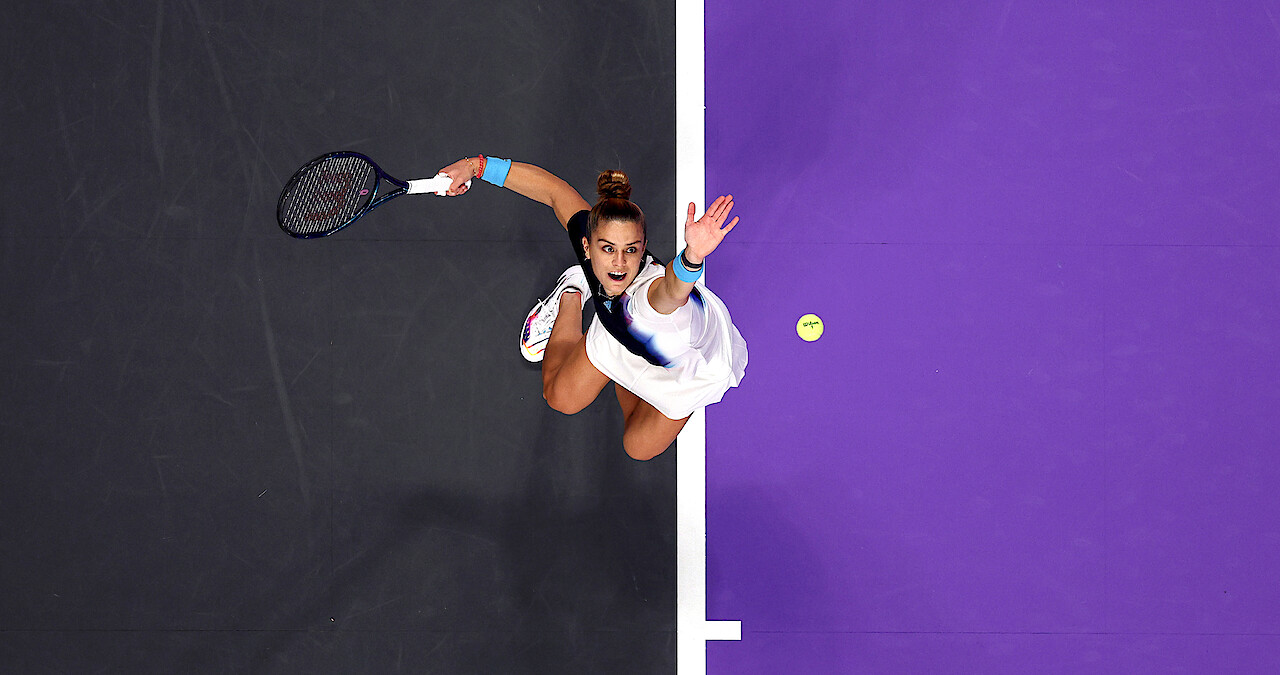
What I Learned
ICP is sensitive to initialization: A good initial guess is crucial for ICP to converge to the correct solution.
Point cloud filtering is essential: To keep the map size manageable and improve performance.
SLAM is hard: But also really cool. Even a basic implementation can give you a sense of how powerful this technology is.