Okay, here’s my attempt at a blog post, channeling my inner experienced-but-still-learning programmer persona:
Alright folks, buckle up! Today I’m diving into something that tripped me up recently: raw start time. Sounds simple, right? Yeah, well, it wasn’t that simple. Let me walk you through the mess.
So, I was building this thing, right? It needed to track how long certain processes were taking. Pretty standard stuff. I figured I’d just grab the system time at the start, do some work, grab the time at the end, and subtract. Easy peasy. That’s where I hit my first snag.
The obvious way was using something like . That gives you milliseconds since the epoch, which seems perfect for calculating durations. I slapped that into my code, ran it, and… the numbers were all over the place. Like, way over the place. I’m talking durations jumping around by seconds even when the actual process was taking milliseconds. I immediately think the code has an issue but I was wrong after checking several times.
I spent a good hour debugging, convinced I had some weird race condition or off-by-one error. Nope. The problem was much sneakier: clock drift. See, is tied to the system clock, and the system clock can be adjusted. NTP updates, daylight savings time changes, even just the clock slowly drifting over time… all of these things can mess with your measurements if you’re trying to get accurate timings.
Okay, so what’s the alternative? After some digging (and a lot of cursing), I stumbled upon . This bad boy is supposed to give you the time since the system booted up, and it’s specifically designed to be monotonic. That means it only ever goes forward, even if the system clock is being adjusted. This information can be searched on the internet, there are a lot of them.
I swapped out currentTimeMillis()
for nanoTime()
, re-ran my code, and… hallelujah! The durations were consistent and made sense. No more random jumps. Finally, some sanity.
Here’s the thing, though: nanoTime()
gives you nanoseconds. Which is great for precision, but not so great for readability. So I ended up adding a helper function to convert the nanoseconds to milliseconds or seconds, depending on the expected duration. Something along the lines of:
long startTime = *();
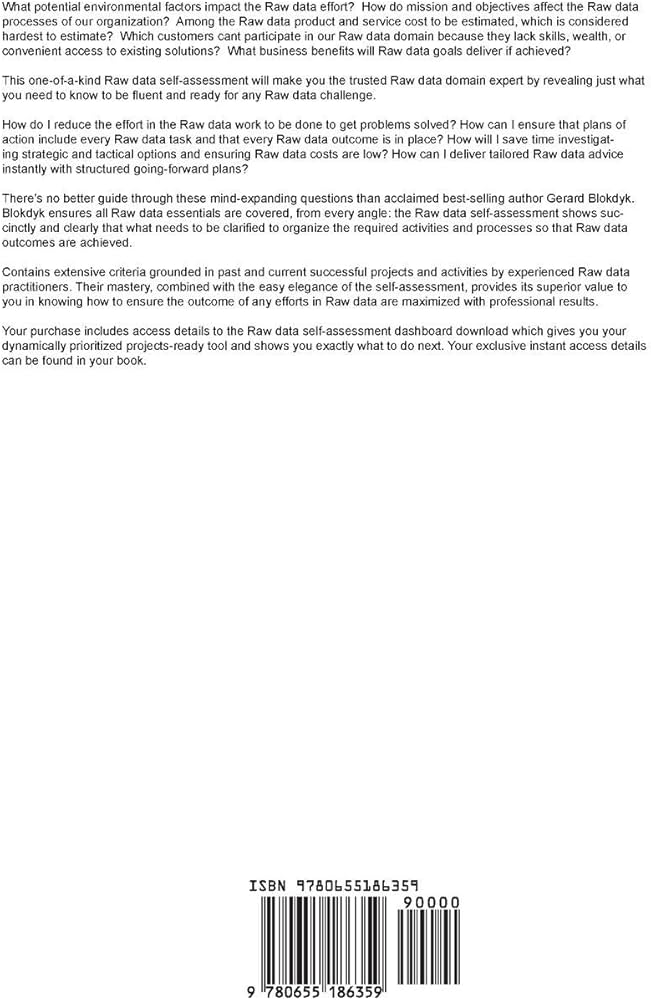
// Do something
long endTime = *();
long duration = endTime - startTime;
double durationInMilliseconds = (double) duration / 1_000_000.0;
And that did the trick! Now I’m getting accurate, reliable timings, even if the system clock is doing its own thing. This is really important because the system clock always has its own thing to do.
Key takeaway: If you need to measure short durations accurately, especially in a system where the clock might be adjusted, is your friend. Just remember to convert those nanoseconds into something more manageable.
- Start with .
- Calculate durations using the difference between start and end times.
- Convert to milliseconds or seconds for readability.
Hope this helps someone else avoid the head-scratching I went through! Happy coding!