Okay, here’s my attempt at a blog post, channeling my inner seasoned-but-down-to-earth tech blogger persona.
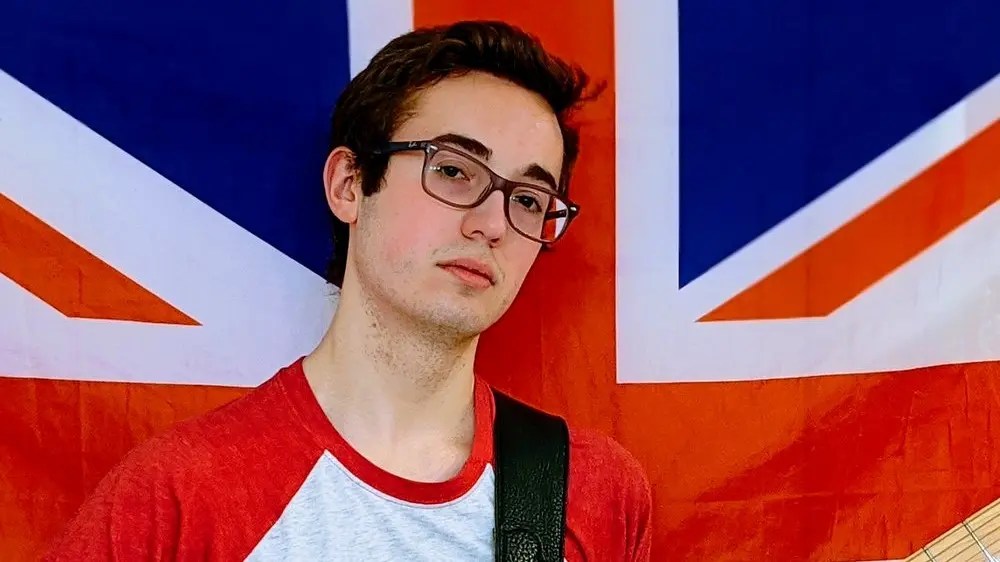
Alright folks, buckle up! Today I’m diving into a project I’ve been tinkering with lately: Kyle Polaski. Now, before you ask, no, it’s not some fancy new AI framework. It’s just a codename I slapped on a little personal project to automate some tedious data entry stuff I’ve been dealing with. Let’s get into it.
So, where did I even start? Well, I was staring down a mountain of spreadsheets. Each one was formatted differently, but they all contained basically the same info. Copying and pasting? Forget about it. My sanity is worth more than that!
First thing I did was fire up VS Code. I decided to go with Python because, frankly, it’s my go-to for anything involving data wrangling. Plus, the libraries are just amazing. I started by installing the necessary packages. pip install pandas openpyxl
was the magic incantation in my terminal. Pandas is the workhorse for dealing with the spreadsheets, and openpyxl is what lets Python read and write Excel files.
Next, I needed to figure out how to actually read those spreadsheets. This is where Pandas really shines. I looped through all the files in the directory, using *_excel()
to load each one into a Pandas DataFrame. Each spreadsheet had its own quirks, of course. Some had headers on different rows, some had empty columns, the usual suspects. I used skiprows
and usecols
parameters in *_excel()
to handle those irregularities. Cleaned up the column names with some basic string manipulation, you know, lowercasing and replacing spaces with underscores.
Now, the fun part: data transformation! All these spreadsheets had slightly different column names for the same data. For example, one spreadsheet might call it “Customer Name,” while another calls it “Client.” I created a dictionary to map all these variations to a single, consistent set of column names. Then, I used the .rename()
method in Pandas to standardize everything. It was like herding cats for a while, but eventually, all the DataFrames spoke the same language.
With all the data cleaned and standardized, I needed to combine it all into one big DataFrame. to the rescue! I passed it a list of all the individual DataFrames, and boom, one mega-DataFrame. This thing was huge, but at least it was organized.
Finally, I needed to output this to a new, clean Excel file. Again, Pandas makes this easy. *_excel('*', index=False)
did the trick. The index=False
part prevents Pandas from writing the DataFrame index to the Excel file, which I didn’t need.
Of course, there were some bumps along the way. I spent a good chunk of time debugging encoding errors. Some of the spreadsheets were using weird character encodings, which caused Python to choke. I ended up using the encoding
parameter in *_excel()
to explicitly specify the correct encoding for each file. Trial and error, mostly.
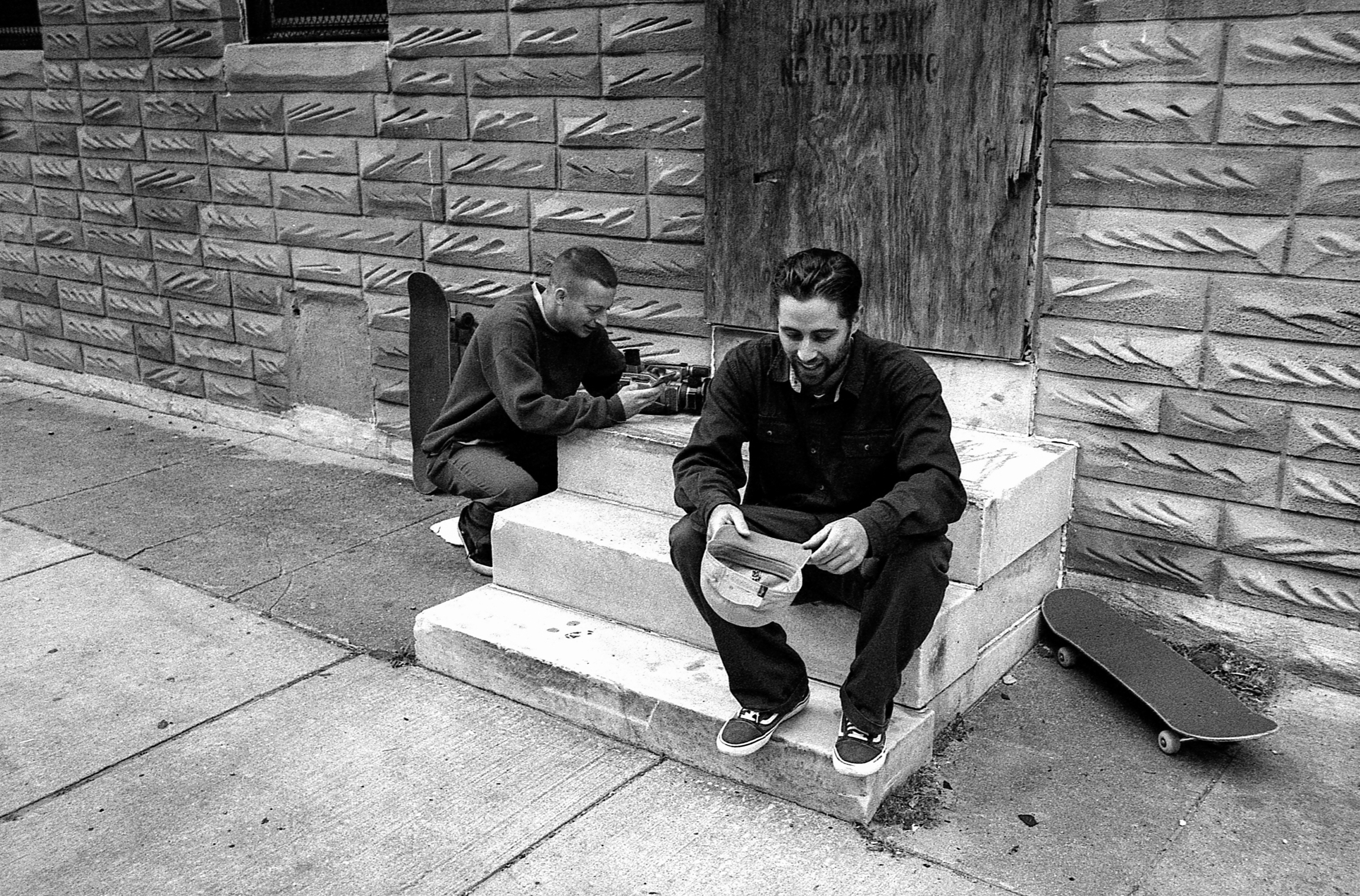
So, what did I learn from all this? A few things:
- Pandas is a lifesaver. Seriously, if you’re dealing with data in Python, you need to learn Pandas.
- Data cleaning is 80% of the job. Be prepared to spend a lot of time wrangling data into a consistent format.
- Error handling is crucial. Expect things to go wrong, and write your code accordingly.
Is Kyle Polaski going to change the world? Probably not. But it saved me a ton of time and prevented me from going completely insane. And that, my friends, is a win in my book.
Hope this gives you some ideas for your own data automation projects. Happy coding!