Alright, let’s talk about this ajax prediction thing I tinkered with. It wasn’t for anything super critical, more like a feature I saw on bigger sites and thought, “Hey, I wonder if I could get that working on my own little playground project.” You know, the typeahead or autocomplete stuff you see in search bars.
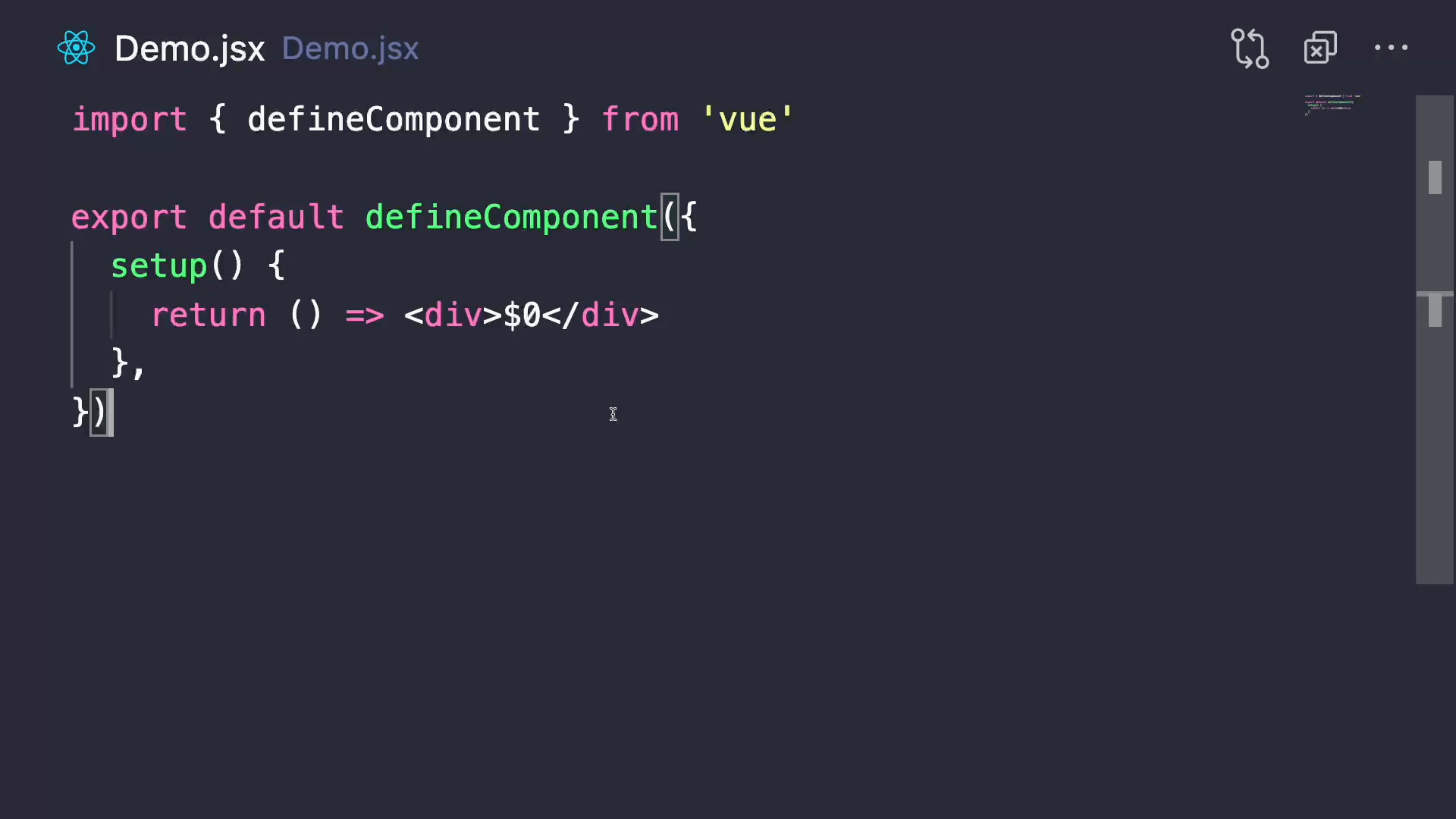
Getting Started
So, the first thing I did was set up a really basic HTML page. Just needed an input box, a plain old <input type="text" id="search-box">
. That was the easy part, obviously. I figured I’d need a place to show the suggestions too, so I added an empty <ul id="suggestions"></ul>
right below it.
Then I jumped into the JavaScript side. I needed something to happen whenever someone typed into the box. I attached an event listener, specifically for the ‘keyup’ event, to that search box. So, every time a key comes up, my function would fire.
Making the AJAX Call
Inside that function, the core idea was to grab the current text from the input box. Simple enough: *('search-box').value
. If the box wasn’t empty, I needed to send this text off somewhere to get predictions.
This is where the AJAX part came in. I used the standard XMLHttpRequest
object. Yeah, I know there are newer ways, libraries and stuff, but I wanted to understand the raw mechanics first. So, I created the request object, opened a ‘POST’ request to a backend script I quickly whipped up (just a simple PHP file for this test), and set the right headers for sending form data.
Here’s a hurdle I hit almost immediately: Every single keypress was firing an AJAX request! If someone typed “hello” quickly, that was five separate requests hitting my backend script. Seemed really wasteful and could potentially slow things down if the backend logic was heavier. So, I had to figure out how to delay it. I wrapped my AJAX call logic inside a setTimeout
. The idea was, after a keypress, wait maybe 300 milliseconds. If another key was pressed within that time, cancel the previous timer and start a new one. Only if the user paused typing for that short duration would the request actually go out. Made things much saner.
Backend and Display
My PHP script was super basic initially. It received the text sent via POST. I had a predefined array of possible prediction strings, like [‘apple’, ‘apricot’, ‘banana’, ‘blueberry’, ‘blackberry’]. The script just looped through this array, checked if any string started with the text I sent, collected the matches, and then spat them back out, usually encoded as JSON.
Back in the JavaScript, inside the AJAX request’s success handler (the bit that runs when the server responds), I parsed the JSON response. This gave me an array of suggestion strings. Then, I cleared out the existing suggestions list (that <ul id="suggestions">
) and dynamically created new <li>
elements for each suggestion received, adding them to the list so they appeared below the search box.
Refinements and Thoughts
It worked! Typing ‘ap’ brought up ‘apple’ and ‘apricot’. Felt pretty neat seeing it function. Of course, it was basic.
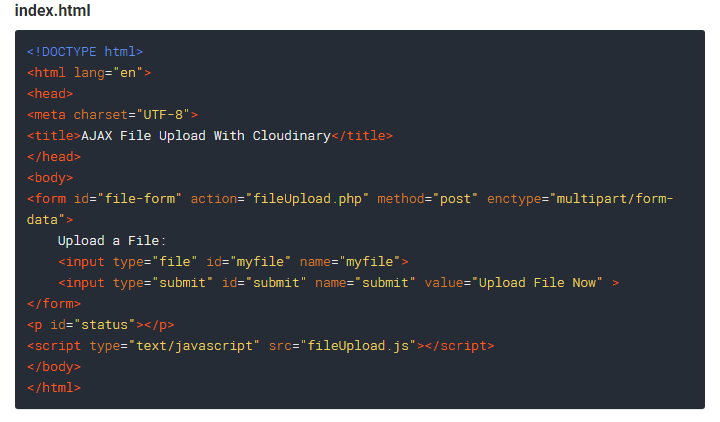
- The prediction list was hardcoded. For a real application, this would obviously need to come from a database or a more sophisticated source. I later changed the PHP to read from a text file, line by line, which was a small step up.
- Error handling was minimal. What if the AJAX call failed? I added some basic console logs, but proper user feedback would be needed.
- Styling the suggestion list took a bit of fiddling with CSS to make it look like a dropdown and position it correctly under the input box. That’s always more time-consuming than you think.
- Accessibility could be improved – adding keyboard navigation for the suggestions, ARIA attributes, etc. Didn’t get to that in this initial run.
But overall, getting that basic AJAX prediction loop working – type, wait a bit, send request, receive data, display suggestions – was a good learning exercise. It really showed the asynchronous nature of web stuff. You type, and other things happen in the background without freezing the whole page. Pretty fundamental stuff, but satisfying to build from scratch, even in a simple way.