Okay, let’s dive into this Ajax thing. I found myself needing to update parts of a page without that annoying full refresh. You know, grabbing some fresh data or sending a quick update to the server. People kept throwing the term “Ajax” around, but there seemed to be a couple of ways folks were doing it.
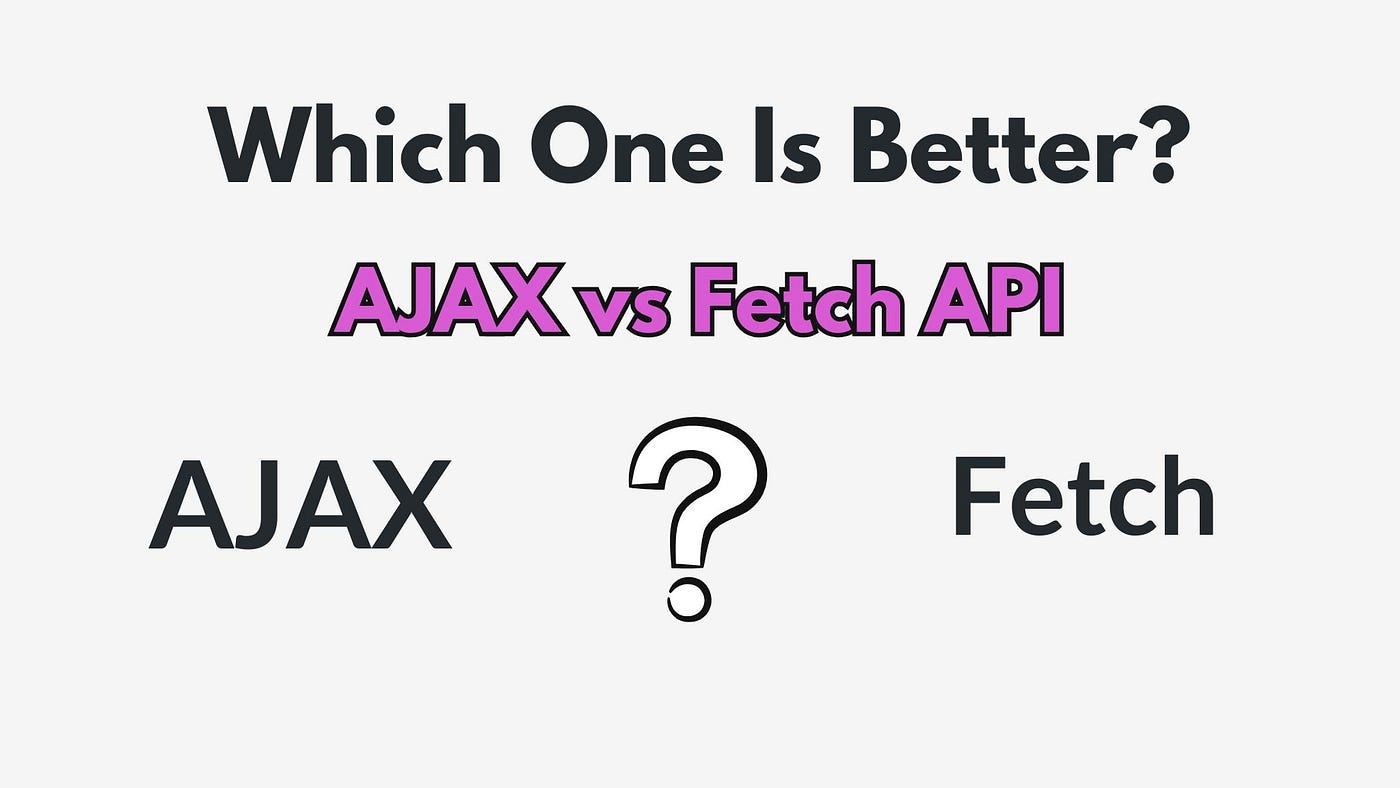
My First Go: The Old School Way
So, naturally, I started with what seemed like the original method: XMLHttpRequest, or XHR. I dug in and started coding. Had to create the XHR object, open a connection, set up this `onreadystatechange` thing to listen for when it was done, and then finally send it off. Honestly, it felt a bit… much. Lots of steps just to fetch a simple piece of data.
I got it working, don’t get me wrong. It pulled the data, updated the page section. But the code looked kinda messy, lots of boilerplate just hanging around. Checking statuses, handling different ready states… felt like I was writing more setup code than actual logic.
Trying Out the Newer Kid: Fetch
Then I kept hearing about fetch. Supposedly simpler, more modern. So, I decided to give it a spin on the same task. Rewrote the little data-grabbing piece using `fetch`. Right away, it looked cleaner. Using Promises (`.then()` and `.catch()`) felt more straightforward than event listeners for state changes.
It went something like this:
- Call `fetch` with the URL.
- Use `.then()` to get the response.
- Use another `.then()` to parse the response (like getting JSON).
- Use `.catch()` for actual network errors.
It wasn’t perfect, though. Took me a minute to realize that `fetch` doesn’t automatically treat server errors (like a 404 or 500) as real errors in the `.catch()` block. You have to check the `*` status yourself inside the first `.then()`. A bit weird at first, but manageable once you know.
What About Libraries?
Of course, I’ve used jQuery’s `$.ajax` in the past on other projects. And yeah, it’s super convenient. Handles a lot of the nitty-gritty details for you, easy syntax, lots of options. But for this particular little project, I was trying to avoid adding extra libraries if I didn’t absolutely need them. Pulling in all of jQuery just for a couple of simple calls felt like overkill this time.
Wrapping Up My Thoughts
So, after playing around with both vanilla ways: XHR felt dated and verbose. It works, but it’s just not pleasant to write anymore. Fetch felt much better, more in line with modern JavaScript, even with its little quirks like error handling. For tasks where I’m already using jQuery, `$.ajax` is still super handy. But for new, simple stuff where I want to keep dependencies low? I found myself leaning towards `fetch`. It just felt like the cleaner path forward for my recent work.